In some cases
we need more than one 7 segment display, But we will be having
limited pins to interface, so we cannot use for example 3X8 port pins
for 3 seven segment displays. Therefore the effective approach is
multiplexing the displays. This method takes advantage of POV i.e.
Persistence Of Vision of a human eye, that means a human eye cannot
observe changes that occur rapidly within milliseconds or microseconds.
So here is the secret, we will access LED displays one by one so fast
that a human eye cannot recognize and it appears like we are driving
all the displays simultaneously.
I have built a quickie circuit with only two displays on a breadboard
(same as above circuit, just ignored dis3 and dis4) I have written
the program for only two displays, you can easily modify the program
for more than two displays.
The assembly language source code for this circuit is given below.
The programs is self explanatory. The first program uses polling method
therefore it cannot be used in actual projects since all the
microcontrollers time is utilized for driving displays, whereas second
one uses timer and interrupts, therefore microcontroller can perform
the main required program and simultaneously drive the displays.
we need more than one 7 segment display, But we will be having
limited pins to interface, so we cannot use for example 3X8 port pins
for 3 seven segment displays. Therefore the effective approach is
multiplexing the displays. This method takes advantage of POV i.e.
Persistence Of Vision of a human eye, that means a human eye cannot
observe changes that occur rapidly within milliseconds or microseconds.
So here is the secret, we will access LED displays one by one so fast
that a human eye cannot recognize and it appears like we are driving
all the displays simultaneously.
Figure
below shows the circuit diagram for driving multiple 7 segment
displays. Here all the display inputs are joined together and connected
to a single port of the microcontroller, the display access is
controlled by the transistors connected to the common anode terminals
of the displays.
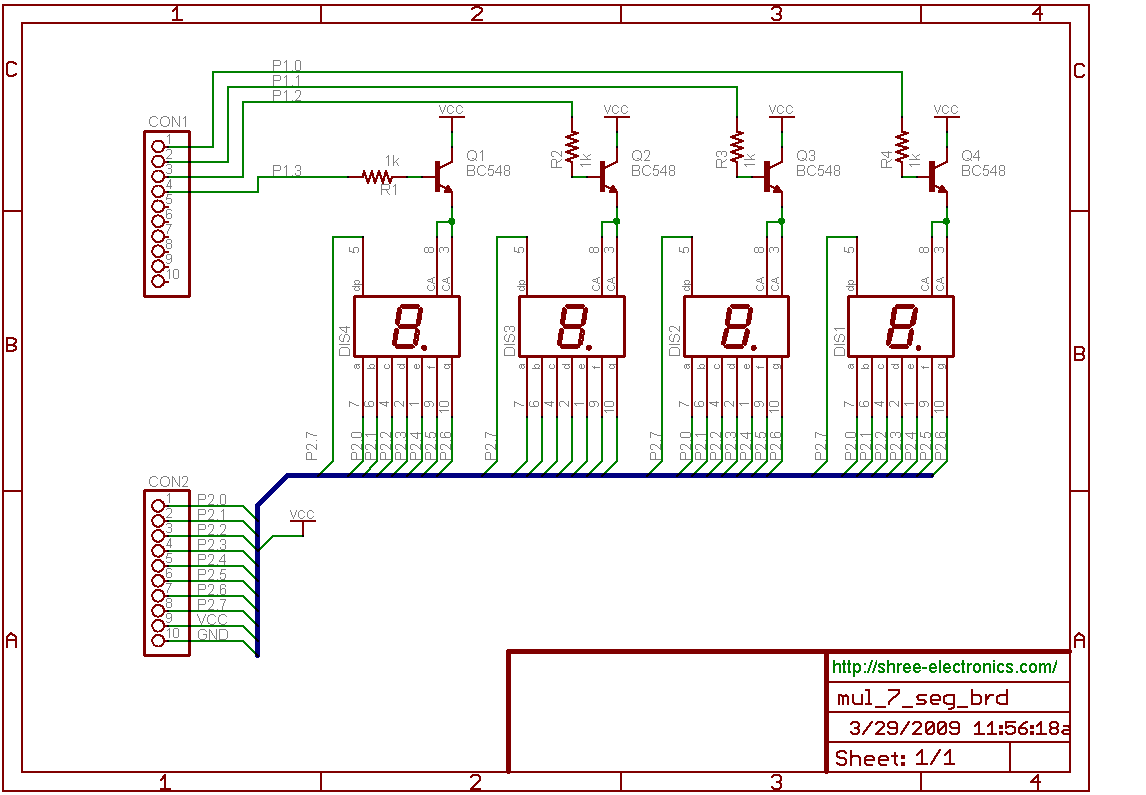
below shows the circuit diagram for driving multiple 7 segment
displays. Here all the display inputs are joined together and connected
to a single port of the microcontroller, the display access is
controlled by the transistors connected to the common anode terminals
of the displays.
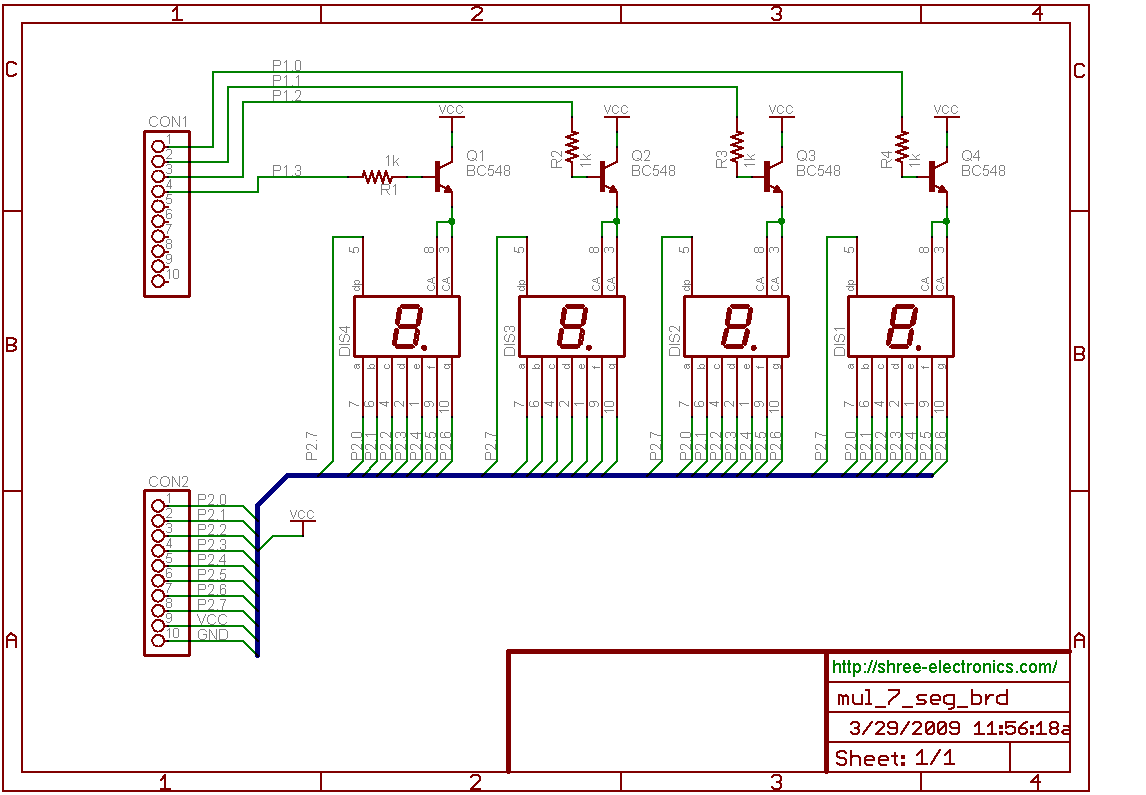
I have built a quickie circuit with only two displays on a breadboard
(same as above circuit, just ignored dis3 and dis4) I have written
the program for only two displays, you can easily modify the program
for more than two displays.
The assembly language source code for this circuit is given below.
The programs is self explanatory. The first program uses polling method
therefore it cannot be used in actual projects since all the
microcontrollers time is utilized for driving displays, whereas second
one uses timer and interrupts, therefore microcontroller can perform
the main required program and simultaneously drive the displays.
|
Using timer and interrupts:
;*************************************************
;
;Program: Driving multiple 7 segment
;Author: Srikanth
;Website: http://shree-electronics.com/
;Description: Displays 0-9 in one display
;and 9-0 in another
;
;*************************************************
;declarations
dis1 equ P1.0;
dis2 equ P1.1;
;*************************************************
;Main program
org 00h
ljmp main
org 0bh ; Timer ISR
ljmp disp
org 30h
main:mov P2,#00h
mov tmod,#01h ;Configure timer
mov TH1,#05h
mov TH0,#0ffh
mov IE,#82h ;Enable timer interrupts
setb TR0 ;start timer
up: mov r2,#11111100b ;0
mov r3,#11111100b ;0
acall delay
mov r2,#01100000b ;1
mov r3,#0F6h ;9
acall delay
mov r2,#0DAh ;2
mov r3,#0FEh ;8
acall delay
mov r2,#0F2h ;3
mov r3,#0E0h ;7
acall delay
mov r2,#066h ;4
mov r3,#0BEh ;6
acall delay
mov r2,#0B6h ;5
mov r3,#0B6h ;5
acall delay
mov r2,#0BEh ;6
mov r3,#066h ;4
acall delay
mov r2,#0E0h ;7
mov r3,#0F2h ;3
acall delay
mov r2,#0FEh ;8
mov r3,#0DAh ;2
acall delay
mov r2,#0F6h ;9
mov r3,#060h ;1
acall delay
sjmp up
;*************************************************
;display subroutine
disp:clr dis1
clr dis2
mov a,r2
cpl a
mov P2,a
setb dis1
mov r4,#0fh
djnz r4,$
clr dis1
mov a,r3
cpl a
mov P2,a
setb dis2
mov r4,#0fh
djnz r4,$
clr dis2
mov TH1,#05h
mov TH0,#0ffh
reti
;*************************************************
;delay subroutine
delay:mov r7,#0ffh
rep:mov r6,#0ffh
rep1:mov r5,#05h
djnz r5,$
djnz r6,rep1
djnz r7,rep
ret
;*************************************************
end